Using the OWASP Top 10 (2021) for Security-Focused Code Reviews
A Practical Guide for .NET Core and Angular Teams
Introduction
Security threats evolve constantly, and leaving application security as an afterthought can lead to costly breaches and reputational damage. To mitigate risk, teams need a structured approach to security during development and one of the most effective ways is to incorporate security checks into code reviews. By using the OWASP Top 10 (2021) as a foundational checklist, your team gains a standardized, well-known reference point for identifying and preventing common vulnerabilities.
This post outlines how to apply the OWASP Top 10 as a code review checklist in a .NET Core and Angular environment. It also suggests integrating automated security tools—such as Semgrep for static application security testing (SAST) and Trivy for software composition analysis (SCA) and secret detection to streamline the process and catch issues early.
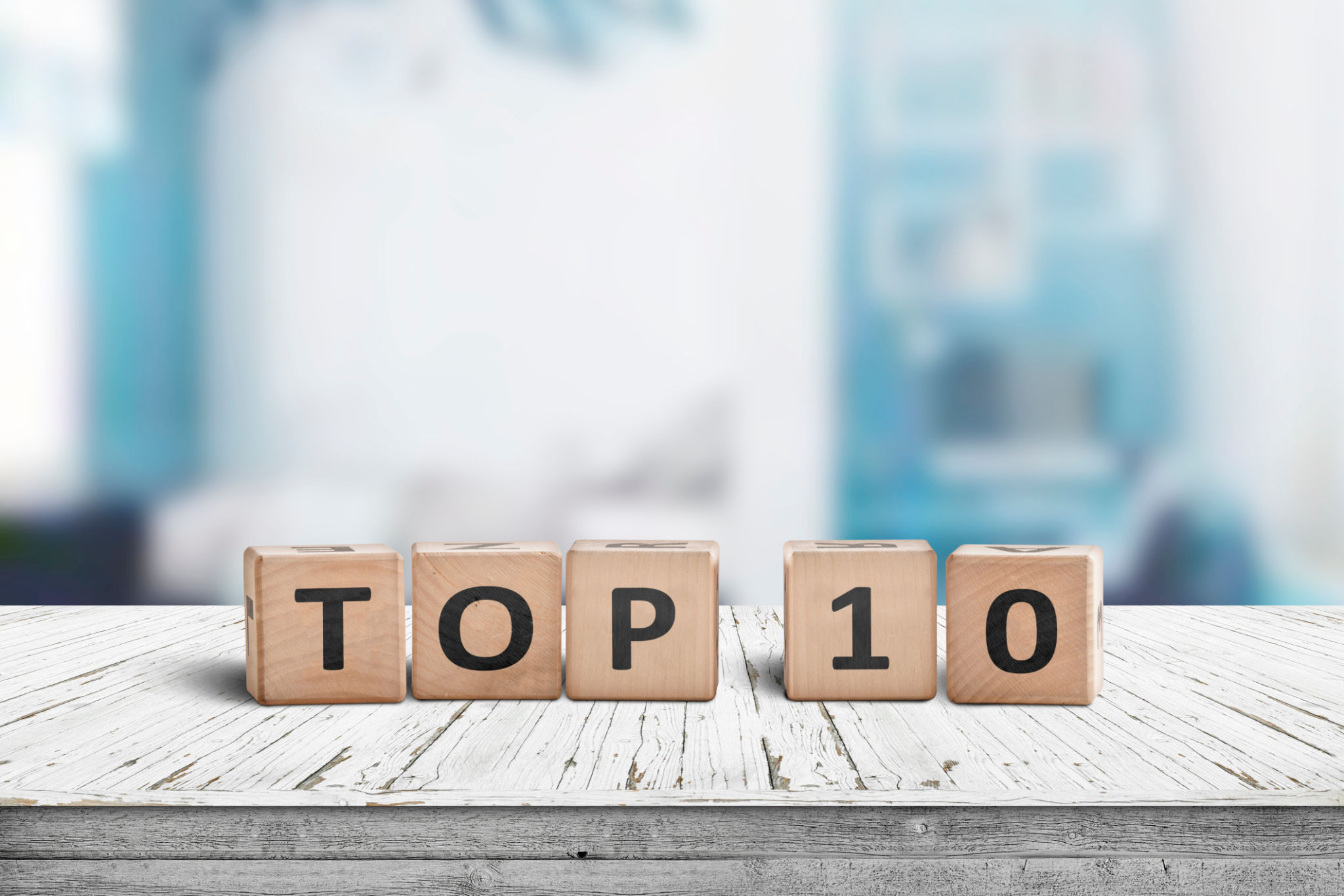
Why Use the OWASP Top 10 During Code Reviews?
The OWASP Top 10 identifies the most critical web application security risks, serving as a baseline for teams looking to improve their security posture. By embedding these checks into your code review process, you ensure that common vulnerabilities are consistently identified and addressed before code merges into your main branch.
When your backend is built with .NET Core and your frontend with Angular, the OWASP Top 10 applies across both layers. .NET Core APIs must be protected against injection, broken access control, and insecure configurations; Angular frontends must prevent cross-site scripting (XSS) and ensure sensitive data is handled securely. Reviewing code with the OWASP Top 10 as a guide ensures that both ends of the stack meet a security baseline.
The OWASP Top 10 (2021) Checklist for Code Reviews
Below are the OWASP Top 10 categories, along with example checks for both .NET Core and Angular applications. Use these as prompts during reviews to ensure nothing slips through the cracks.
(1) Broken Access Control
- .NET Core: Check that [Authorize] attributes are in place for protected endpoints. Validate role-based or claims-based policies thoroughly.
- Angular: Confirm that routing guards (CanActivate) align with server-side authorization logic. Client-side checks should never be the sole source of protection.
Tools: Semgrep can detect missing authorization checks. Trivy can flag vulnerable dependencies that could undermine access control logic.
(2) Cryptographic Failures
- .NET Core: Ensure passwords are hashed and salted (PBKDF2, BCrypt, or Argon2), and review code for proper use of ASP.NET Core Data Protection APIs.
- Angular: Verify that sensitive tokens are not stored insecurely (e.g., not in local storage if avoidable), and that all API calls use HTTPS.
Tools: Semgrep can identify weak crypto usage patterns. Trivy can detect outdated TLS libraries or insecure crypto dependencies.
(3) Injection
- .NET Core: Confirm that Entity Framework or Dapper queries are parameterized. Avoid dynamic SQL concatenation.
- Angular: Validate and sanitize user input before rendering. Use Angular’s built-in sanitization for dynamic HTML to prevent XSS.
Tools: Semgrep can spot SQL injection patterns. Trivy can detect components with known injection-related vulnerabilities.
(4) Insecure Design
- .NET Core: Review architecture diagrams and code to ensure the design inherently reduces attack surfaces (e.g., layered services, DTOs, no direct DB calls in controllers).
- Angular: Confirm that critical security decisions (like authorization) aren’t solely made client-side.
Tools: Semgrep’s custom rules can flag insecure architectural patterns. Trivy identifies vulnerable components that could weaken the application’s security posture.
(5) Security Misconfiguration
- .NET Core: Check that appsettings.json does not contain secrets in plaintext, and that security headers (HSTS, CSP) are correctly configured.
- Angular: Ensure no debug configurations, verbose logs, or environment files with sensitive data leak into production builds.
Tools: Semgrep rules can detect hardcoded secrets in code. Trivy’s secret detection feature can find credentials mistakenly committed to the repository.
(6) Vulnerable and Outdated Components
- .NET Core: Verify NuGet packages are up to date and monitor for known vulnerabilities.
- Angular: Regularly update npm packages and use Angular’s ng update to stay current.
Tools: Trivy’s SCA capabilities scan csproj and package.json files, flagging vulnerabilities in dependencies.
(7) Identification and Authentication Failures
- .NET Core: Ensure ASP.NET Core Identity enforces strong passwords, account lockouts, and secure session management.
- Angular: Confirm tokens (JWTs) are handled securely, short-lived, and validated server-side.
Tools: Semgrep can detect insecure token validation logic. Trivy ensures no vulnerable identity libraries are in use.
(8) Software and Data Integrity Failures
- .NET Core: Check that you trust and verify external packages, and ensure CI/CD pipelines enforce integrity (e.g., code signing).
- Angular: Use Subresource Integrity (SRI) for external scripts.
Tools: Trivy can detect tampered images or compromised libraries. Semgrep can find insecure file handling patterns.
(9) Security Logging and Monitoring Failures
- .NET Core: Verify that loggers (Serilog, .NET built-in logging) do not record sensitive data like passwords or tokens. Ensure logs are monitored and analyzed.
- Angular: Limit console logging in production.
Tools: Semgrep can detect logging of sensitive information. Trivy can highlight dependencies known to cause logging issues.
(10) Server-Side Request Forgery (SSRF)
- NET Core: Validate any user-supplied URLs before making outbound calls. Apply network segmentation and allowlists.
- Angular: Avoid client-side logic that blindly trusts input to form requests. All validation should happen server-side.
Tools: Semgrep can flag suspicious outbound requests. Trivy identifies dependencies vulnerable to SSRF exploits.
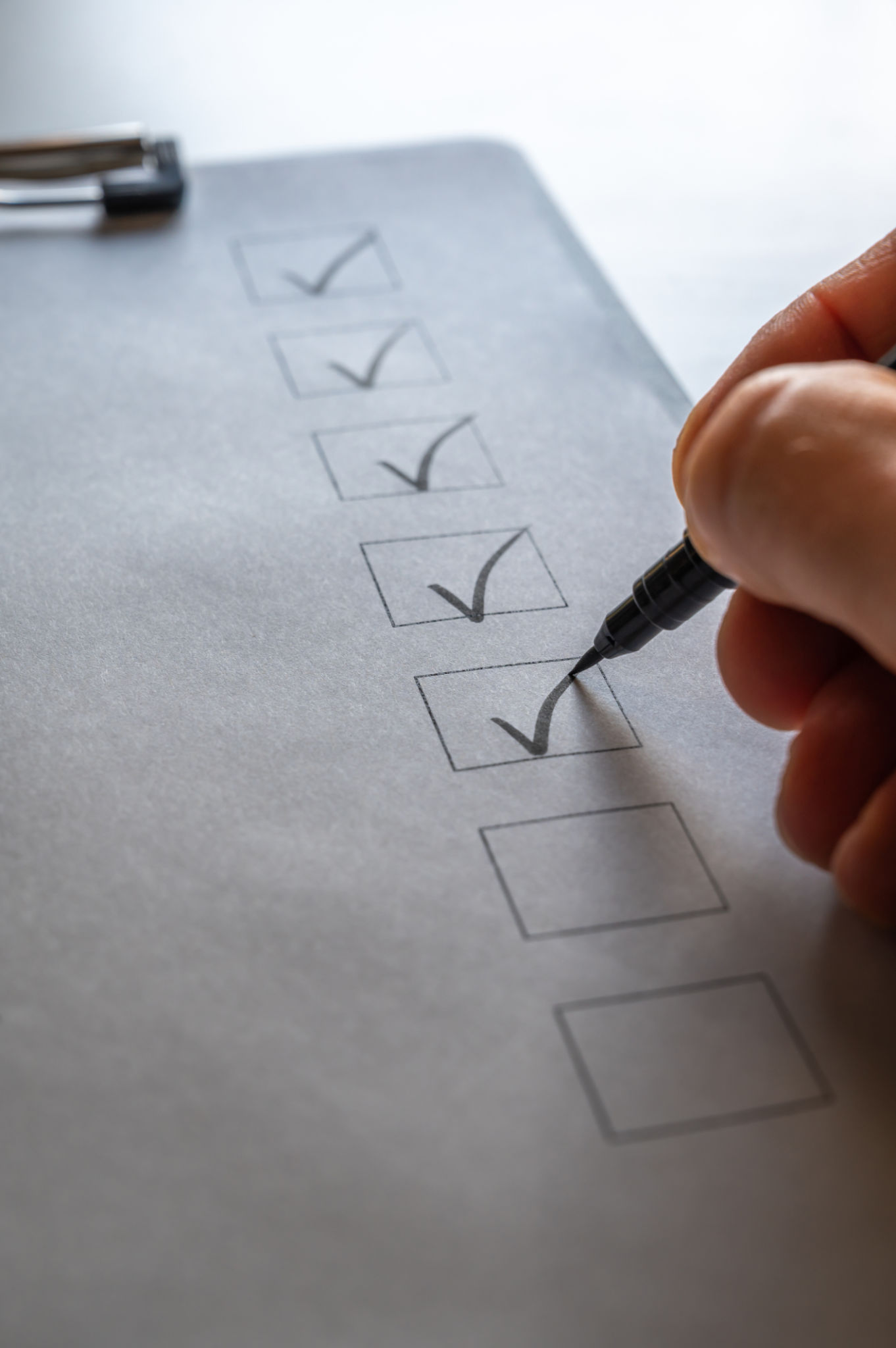
Integrating Semgrep and Trivy Into Code Reviews
Semgrep (SAST):
- Add Semgrep checks into your CI pipeline. Every pull request triggers Semgrep to scan .NET Core (C#) and Angular (TypeScript) code for known security patterns.
- Customize rules based on OWASP Top 10 categories, ensuring high-priority issues—like injection or broken access control—are flagged immediately.
Trivy (SCA & Secret Detection):
- Use Trivy to scan your application’s dependencies, container images, and source code for known vulnerabilities and accidental secret leaks.
- Incorporate Trivy scans into the CI workflow so any introduction of vulnerable or outdated components is caught before merging.
By combining OWASP Top 10 checks with automated tools, you reduce reliance on human memory and ensure consistent application of security standards.
Conclusion
Using the OWASP Top 10 (2021) as a checklist brings clarity and consistency to code reviews. In a .NET Core and Angular environment, these reviews help protect both backend APIs and frontend interactions against common vulnerabilities. Integrating tools like Semgrep for SAST and Trivy for SCA and secret detection further strengthens your security posture by providing automated, continuous vigilance.
Remember, security is an ongoing effort. As your team grows more comfortable applying the OWASP Top 10 during code reviews and leveraging automated checks, you’ll foster a security-first culture—one that delivers safer, more resilient software to users.